Game States: How To
By Boice Wong Rffr
This tutorial will walk you through on creating and using game
states in your program. Game states allow you to manipulate UI and game code
separately. We will now begin.
- Step one: Game states are a very good way
to format when starting to create a game. The only prerequisites are to
have a basic game shell (e.g. Update method, threads, etc..).
- To create the game states, you first need
create your current state. You also need to have a list of game state
enumerations.
The
states we will be using as an example are just BEGIN and GAMEOVER,
however you can create as many game states as necessary.
- Step two: Now you need to go to your
update method and use the switch statement because we are using
enumerations.
- The syntax for the game states within the
switch statement is to type “case” and then your game state. Also make
sure to use a colon to end the line not a semicolon.
- The next line will be where you tell the
program what to do during the gamestate. In the last line you type in
“break;” to let the program know that that is the end of the game state
case. Then you repeat for the rest of your game states as so.
What
is really useful with game states is that you can make methods and then
just call them in the game state. It is really helpful to organize your
program efficiently.
- Step three:
- The last thing to do is to figure out
which gamestate the program is in. To do this, you type, currentState =
GameState.gamestatename; You can do this wherever you want the game to
change states.

- How to reset the game.
- One example of the use of game states is
to determine when the game needs to be reset. In this case, we will use
the example of a brick breaker rebounding game where if you fail to
bounce the ball upwards, you lose and the game will reset.
- The game states are set up as shown
in this tutorial
- One necessary method is the Reset()
method which calls the Initialize method with a global context and also
clears the game. This is how the game resets.
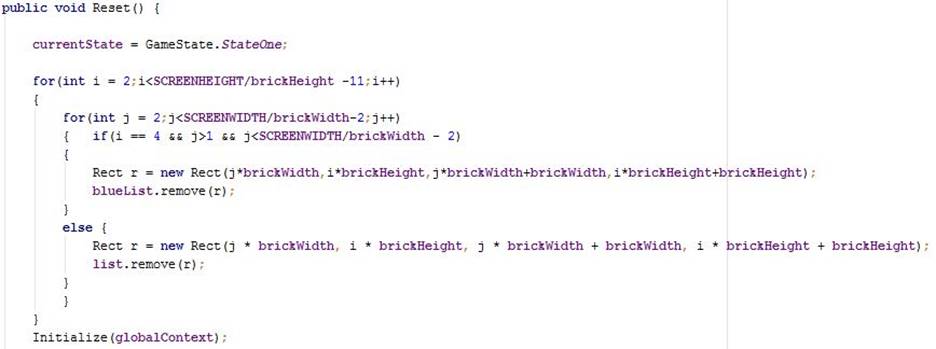
- Once your ball “dies” in the game, a
screen will pop up saying to touch to reset. This program has a
touchpoint listener and when you touch it, the game state will change.
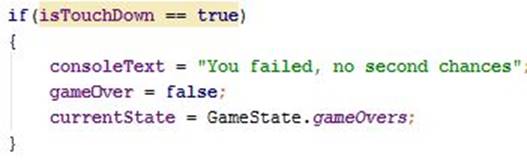
- The game state looks like this
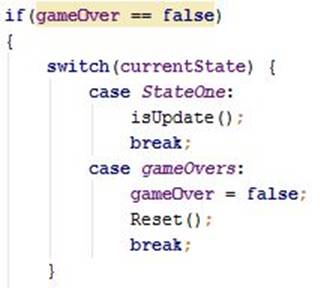
- Since the touch changes the game state to
gameOvers, the Reset() method is called which changes the game state back
to StateOne.
- Now go and try it yourself!